Fun with Hardcoded Secrets
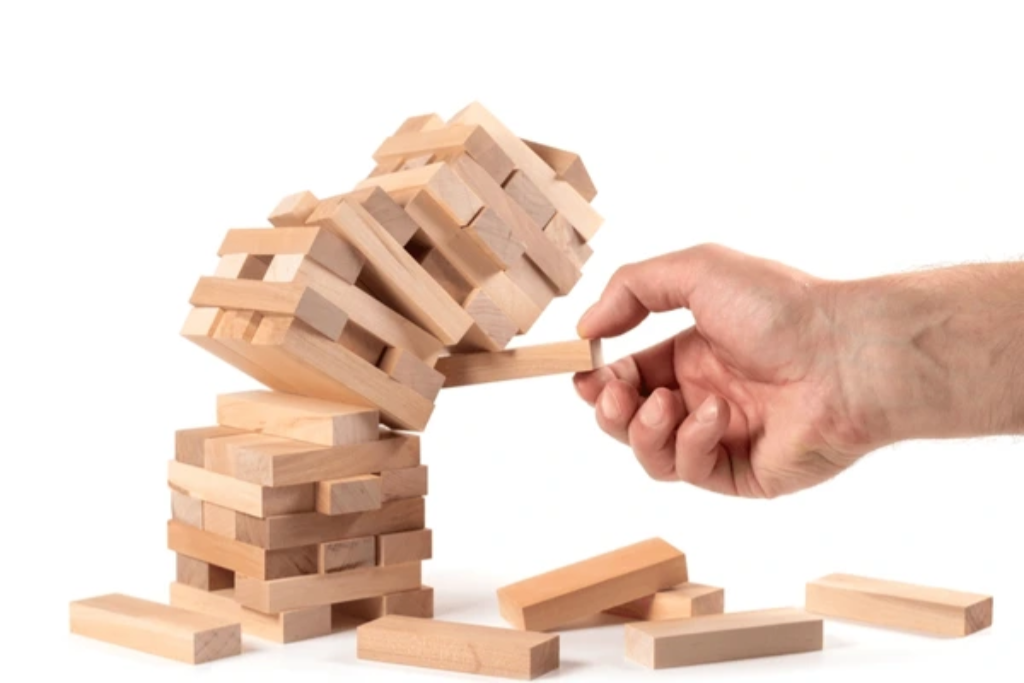
I was doing some consulting work for a company a few years back and came across a hardcoded secret in their source code. Being the sort that likes to pull on loose threads I decided to see where it would lead - and it turned out that the resulting vulnerability was even more fun than I was expecting.
It all started with a simple hashing function embedded in a library, that went something like this:
public static string CreateHash(string plaintext) { const string salt = "BLAHBLAHBLAHBLAH"; return GenerateHash(plaintext, salt); }
Now this might not look all that bad - it's just a hash function that uses a hardcoded salt.... and depending on how, and where, it was being used it might not even cause any problem.
But in this case the company was a service provider that created custom websites for its customers and this function was in a shared library that was used in all of the websites that they built - each time they got a new customer, they would copy their current codebase into another repository and then start customizing it to match their customer's specifications. Since the salt value was hardcoded in the library, every customer's website would then generate the same hash values for whatever strings were run through the hashing function.
So here's where it started to get bad - the company also gave their clients an administrator portal that allowed system administrators to login to these websites and impersonate one of their site's end users. In the administrative portal, an admin could select a user, then click a button titled something like "Logon as User" and they would be redirected to a URL on the website that took a token as a query parameter and would then let the admin logon to the website as though they were the user associated with that token... unfortunately, the programmers who built this functionality didn't have much experience with computer security and so they implemented this in the worst possible way.
Now the right way to implement something like that could be really simple and still reasonably secure - for instance, when the admin clicks the "Logon as User" button you might generate a one-time random token, store it in the database, and then when you redirect to the web site that site compares the token you provide to the one in the database, logs you in as the user, and then of course the system would delete that token so that it couldn't be reused. But that's not what happened here, instead the system generated the impersonation token for each user at the time the user's account was created and then stored that token along with the rest of the user's information. I bet you can guess how they created the impersonation token right? You guessed it - they ran the user's email address through the secret hash function and then stored the resulting hash as the impersonation token.
So this is now a serious problem – because if someone at Customer A knows that the secret hash function generated for "bob@example.com" on their web site will produce the same hash that's used at Customer B's site, they can now impersonate any one of their competitor's customers simply by creating a corresponding account in their own system using that customer's email address.
But if you thought that was bad, it's about to get a lot worse. Right now we have a situation where one customer can use insider knowledge to access a different customer's web site, which is bad enough, but a little more digging turned up something even more entertaining:
The customer web sites also allowed their end users to upload profile pictures that would be associated with their account on the web site and other users on that web site could see those profile pictures. Do you want to guess how the system generated the filenames for the profile pictures when they were uploaded?
Yep, they ran the user's email address through the secret hash function and then stored the file using the resulting hash as the filename.
And the final nail was that none of these websites used email verification, so any random person could create an account on any of the customer web sites using whatever email address they pleased.
So if you know "bob@example.com" is an end user on Customer A's web site, all you have to do is go to Customer B's web site, create a new account using the same email address and then upload a profile picture. The resulting URL of your shiny new profile picture on Customer A's web site is also the impersonation token for "bob@example.com" on Customer B's web site.
It's hard to believe you can still run into vulnerabilities like this in modern code bases but they're out there and this is a good example of why you need to ensure that you have security reviews built in to your development process.