Programmatically Deploying Wordpress Sites Using Docker and Node.js
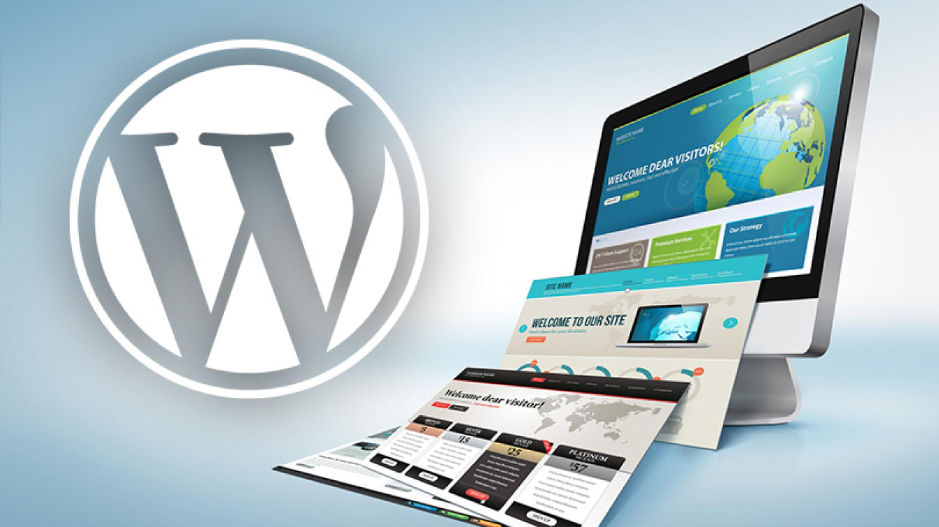
In this article I'm going to show you how to programmatically generate instances of a Wordpress site using Node.js and Docker Compose. This is useful if you'd like to automate the process of provisioning individual blogs for your end users or spinning up separate application sites, etc.
Note: For the sake of keeping this tutorial simple, we're going to assume that everything will be running on the same server.
1. Docker Compose
The first part of our solution is going to be developing a template for a docker compose file that we can use to automatically install a stand alone instance of Wordpress. The resulting container will include it's own web server, MySQL database, and Wordpress installation. Since we'll need to customize the file for each new user that we create a new site for, we'll write this as a LiquidJS template and then fill in the variables later.
services:
db:
container_name: {{account_id}}-db
image: mariadb:10.6.4-focal
command: '--default-authentication-plugin=mysql_native_password'
volumes:
- /var/www/sites/{{account_id}}/mysql_data:/var/lib/mysql
restart: always
environment:
- MYSQL_ROOT_PASSWORD={{mysql_root_password}}
- MYSQL_DATABASE={{mysql_site_database}}
- MYSQL_USER={{mysql_site_username }}
- MYSQL_PASSWORD={{mysql_site_password}}
expose:
- 3306
- 33060
wordpress:
container_name: {{account_id}}-wp
image: wordpress:latest
ports:
- 80
restart: always
volumes:
- /var/www/sites/{{account_id}}/wordpress:/var/www/html
environment:
- WORDPRESS_DB_HOST=db
- WORDPRESS_DB_USER={{mysql_site_username}}
- WORDPRESS_DB_PASSWORD={{mysql_site_password}}
- WORDPRESS_DB_NAME={{mysql_site_database}}
depends_on:
- "db"
wpcli:
container_name: {{account_id}}-cli
image: wordpress:cli
user: 33:33
depends_on:
- "wordpress"
command: tail -f /dev/null
volumes:
- /var/www/sites/{{account_id}}/wordpress:/var/www/html
environment:
WORDPRESS_DB_HOST: {{account_id}}-db
WORDPRESS_DB_USER: {{mysql_site_username }}
WORDPRESS_DB_PASSWORD: {{mysql_site_password}}
WORDPRESS_DB_NAME: {{mysql_site_database}}
Now that we have a template that can be used to generate a container for MySQL, WordPress, and the WordPress Command Line Interface (WP CLI), the next thing we need to do is create a template for a reverse proxy entry that we can use to add any new WordPress site that we create to our web server. Each WordPress site that we will create is going to have it's own special subdomain, like "mysite.{somedomain}.com" and will be running on it's own non-standard port, so we will have to create a new virtual server entry in our web server's configuration each time we create a new site so that requests are routed correctly.
server {
listen 80;
listen [::]:80;
server_name {{subdomain}}.{{host}};
location / {
proxy_set_header X-Forwarded-Host $host:$server_port;
proxy_set_header X-Forwarded-Server $host;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header Host $host;
proxy_pass http://{{subdomain}}.{{host}}:{{port}};
}
}
Okay, so after we have a created Docker Compose template for generating our containers, and an NGINX template for creating the corresponding reverse proxy entries, let's put together a simple node.js API that we can call to generate a new WordPress instance. Since we're keeping this tutorial simple, we're just going to say that this API will be present on the same host were we are creating the containers, so that it's easy for it to create directories, write files, etc.
The API will be located at "/sites" off of the main URL and we can post to that API to request that the system generate a new WordPress installation.
The request body should look like this:
{
"account_id": "49c60352-b08f-426b-a25d-5e1b24782f68",
"account_domain": "example.com",
"account_subdomain": "joeuser",
"account_email_address": "joeuser@example.com",
"account_admin_password": "PASSWORD FOR WORDPRESS ADMIN"
}
When the API receives the request, it will create a separate folder using the account identifier that is in the request body, so we have:
/var/www/sites/49c60352-b08f-426b-a25d-5e1b24782f68
As the home directory for the new WordPress installation, and then we will write out the docker-compose.yml file, execute some local docker commands to finish up installing WordPress, then we'll add the reverse proxy information to NGINX, restart the web server, and then we're good to go.
Here's the example API code:
var express = require('express');
var router = express.Router();
const { Liquid } = require('liquidjs')
const uuid = require('uuid')
const path = require('path')
const util = require('util');
const exec = util.promisify(require('child_process').exec);
const fsPromises = require('fs').promises;
router.post('/', async function(req, res) {
// Configure the substitution parameters for the LiquidJS template
// that we will render to generate the docker-compose.yml file for
// deploying the new collection of containers for this user's site:
const configuration = {
account_id: req.body.account_id,
account_subdomain: req.body.account_subdomain,
mysql_site_database: 'wordpress',
mysql_site_username: 'wordpress_user',
mysql_site_password: uuid.v4(),
mysql_root_password: uuid.v4(),
}
// Now that we have all the parameters that we need, let's create a
// folder on the local filesystem that will act as the home directory
// for the new WordPress install; we want both the MySQL container and
// the WordPress container to be able to persist data so that their
// configurations can survive a reboot and so on.
const sitepath = "/var/www/sites/" + configuration.account_id;
try {
await fsPromises.mkdir(sitepath);
} catch(x) {
res.status(500).json({ message: "Couldn't create installation directory"})
return;
}
// Render the docker-compose.yml file and save it in the root of the
// user's site directory:
try {
const engine = new Liquid({root: path.join(__dirname + '/../templates'), extname: '.liquid'});
const rendered_template = engine.renderFileSync('docker-compose', configuration);
await fsPromises.writeFile(sitepath + '/docker-compose.yml', rendered_template);
} catch(x) {
res.status(500).json({ message: "Couldn't create docker-compose file."})
return;
}
// Now let's run docker compose and start all the containers:
try {
// The account_id of the user who owns this site is used as the project name
// for the Docker Compose project. This just makes it easier to find and manage
// the resources associated with a particular user's account.
const project_name = configuration.account_id;
// The user's installation gets it own instance of the docker-compose.yml file:
const project_file = sitepath + "/docker-compose.yml";
// And now we just run Docker Compose to startup the containers:
await exec("docker compose -f " + project_file + " -p " + project_name + " up -d");
// After Docker Compose has started all the containers, we want to get the
// specific port that Docker has exposed on the WordPress container:
const { stdout } = await exec("docker port " + project_name + "-wp");
// extract the internal port number from the resulting string
const wordpress_container_expr = /(\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}):(\d{1,5})/
const wordpress_container_port = wordpress_container_expr.exec(stdout)[2];
// Now we can use the WordPress CLI container to adjust the configuration
// in the WordPress container:
let init_wp_command = "docker exec " + req.body.account_id + "-cli wp core install";
init_wp_command = init_wp_command.concat(" --url=" + req.body.account_subdomain + "." + req.body.account_domain);
init_wp_command = init_wp_command.concat(" --admin_user=" + req.body.account_email_address);
init_wp_command = init_wp_command.concat(" --admin_password=" + uuid.v4());
init_wp_command = init_wp_command.concat(" --title='Just Another WordPress Site'");
init_wp_command = init_wp_command.concat(" --admin_email=" + req.body.account_email_address);
// NOTE: It takes a second for the WordPress container to finish
// initializing, so if we try to use the WP CLI container right
// now it will fail. This is fine for a demo, but you'd need a better
// mechanism if you were going to do this in production.
await new Promise(r => setTimeout(r, 5000));
// Execute the WP CLI command to complete the WordPress installation:
await exec(init_wp_command);
// Now we're going to create a new reverse proxy configuration for this
// installation and add it to the NGINX server's available-sites:
const virtual_host_configuration = {
host: req.body.account_domain,
port: wordpress_container_port,
subdomain: req.body.account_subdomain
}
const rendered_virtual_host_entry = engine.renderFileSync('nginx-virtual-host', virtual_host_configuration);
// Append to the end of the NGINX virtual hosts file:
const virtual_hosts_file = '/etc/nginx/sites-available/wordpress_sites'
await fsPromises.appendFile(virtual_hosts_file, rendered_virtual_host_entry, { flag: "as"});
// Restart NGINX:
await exec("systemctl reload nginx");
} catch(x) {
res.status(500).send(x);
return;
}
res.status(201).send("Your wordpress site is ready.");
});
You can download the updated and tested example code for this from my GitHub account at: